The Raspberry Pi can be used to send 433MHz signals to other devices. Here we show how to connect a Raspberry Pi to a transmitter module to send RF signal codes.
Table of contents
Introduction to 433MHz RF communication from a Raspberry Pi
Radio waves can be used as a form of wireless communication between two devices. 433MHz is a commonly used frequency band to communicate between devices that require little power. Devices include remote controls, headphones, baby phones, and many more.
The Raspberry Pi can also be used to send and receive radio frequency (RF) signals by connecting its GPIO pins to a 433MHz RF Transmitter Receiver module.
This post will show how to connect the Raspberry Pi to a 433MHz RF Transmitter module and send RF signal codes to a Receiver module using Bash or Python. In this case, the Receiver module will be connected to an Arduino, but a Raspberry Pi can also be used.
- Get the Raspberry Pi 4B 4GB Starter Kit from Amazon.com
- Get the Raspberry Pi 4B 8GB Starter Kit from Amazon.com
- Get the Basic Arduino Uno R3 Starter Kit from Amazon.com or BangGood
- Get the 433Mhz RF Transmitter Receiver Module Kit from Amazon.com or BangGood
Requirements & assumptions
The Raspberry Pi will need the Raspberry Pi OS (formerly known as Raspbian) as the operating system. Initially, a connection to the internet will be needed to install the required libraries. The command line, Bash or a Python script will be used to send the RF signals.
If you don’t have a screen, keyboard, and mouse you will need to be on a network and use PuTTY and/or WinSCP for the setup, sending of signal codes, testing, and coding.
To prepare the Arduino for receiving signals, it will need to be connected to a computer and the Arduino IDE with the serial monitor activated. Any Arduino can be used.
You will also need:
- 2 pairs of 433 MHz Transmitter Receiver units Amazon.com or BangGood
- 433 MHz Spiral Spring Helical Antennas (optional), but will help to extend the range between communicating units Amazon.com or BangGood
- Solderless developing breadboard and some additional breadboard wiring (Arduino and Raspberry Pi compatible) Amazon.com or BangGood
Setting up the Raspberry Pi to transmit RF signals
To set up the Raspberry Pi to transmit RF signals, it needs to be connected to the 433MHz Transmitter module. The Raspberry Pi (Raspbian) also needs to be prepared and configured using wiringPi and a modified version of 433Utils.
Connecting the Raspberry Pi to a 433MHz Transmitter module
The 433MHz RF transmitter module is the square (vs. rectangle) module of the 433MHz RF Transmitter Receiver module pair.
The transmitting range of the Transmitter module can be extended by adding an antenna. The official 433 MHz Spiral Spring Helical Antennas can be used, or one can be made by coiling a 25 cm 1 mm solid wire and soldering it into the designated antennae hole.
When positioning the Raspberry Pi GPIO pins facing up and on the opposite side, i.e. with the USB and Ethernet port on the right, the physical GPIO pin numbering will be as follows:
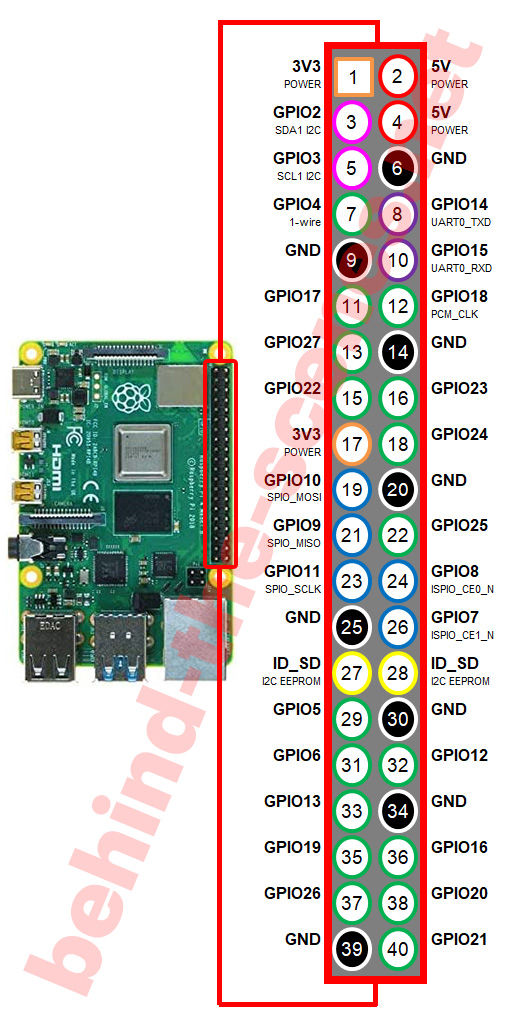
GPIO pins of the Raspberry Pi. These are used to connect to a transmitting 433MHz Transmitter module to communicate RF signals from a Raspberry Pi.
The pins on the 433MHz RF Transmitter module are clearly marked.
The Transmitter module is connected to the Raspberry Pi as follows:
- ATAD (DATA) to physical GPIO pin number 11 (i.e. GPIO17)
- VCC (5 V) to physical GPIO pin number 2 or 4
- GND to physical GPIO pin numbers 6, 9 or 14
Preparing the Raspberry Pi to transmit 433MHz RF signals
After the Transmitter module has been connected, Raspbian needs to be prepared. For this, wiringPi and a modified version of 433Utils can be used. Both are installed using terminal commands.
For the purpose of this post, the home/pi
directory will be used as the project directory. Any other directory can also be used.
First, the Raspberry Pi should be updated and upgraded to the latest version:
sudo apt-get update sudo apt-get upgrade
wiringPi is cloned using the git
terminal command:
sudo apt-get install git
The following commands can be used from the /home/pi
directory:
cd /home/pi git clone git://git.drogon.net/wiringPi cd wiringPi ./build
This will automatically create /wiringPi
in the home/pi
directory.
After wiringPi is installed, the build can be verified by using the gpio readall
command:
gpio readall
which, if correctly installed, will give the state of each pin on the GPIO:
+----------+-Rev1-+------+--------+------+-------+ | wiringPi | GPIO | Phys | Name | Mode | Value | +----------+------+------+--------+------+-------+ | 0 | 17 | 11 | GPIO 0 | OUT | Low | | 1 | 18 | 12 | GPIO 1 | IN | Low | | 2 | 21 | 13 | GPIO 2 | IN | Low | | 3 | 22 | 15 | GPIO 3 | IN | Low | | 4 | 23 | 16 | GPIO 4 | IN | Low | | 5 | 24 | 18 | GPIO 5 | IN | Low | | 6 | 25 | 22 | GPIO 6 | IN | Low | | 7 | 4 | 7 | GPIO 7 | IN | Low | | 8 | 0 | 3 | SDA | IN | High | | 9 | 1 | 5 | SCL | IN | High | | 10 | 8 | 24 | CE0 | IN | Low | | 11 | 7 | 26 | CE1 | IN | Low | | 12 | 10 | 19 | MOSI | IN | Low | | 13 | 9 | 21 | MISO | IN | Low | | 14 | 11 | 23 | SCLK | IN | Low | | 15 | 14 | 8 | TxD | ALT0 | High | | 16 | 15 | 10 | RxD | ALT0 | High | +----------+------+------+--------+------+-------+
Note how the physical GPIO pin numbers change. Physical GPIO pin number 11, for example, becomes GPIO 0 with wiringPi.
To install (clone) 433Utils onto the Raspberry Pi, go back to the /home/pi
directory and use the git clone
command again:
cd /home/pi git clone https://github.com/ninjablocks/433Utils.git
This will automatically create /433Utils.RPi_utils
in the home/pi
directory. RF signals are sent while inside the /433Utils.RPi_utils
directory using codesend.cpp
.
cd 433Utils/RPi_utils sudo nano codesend.cpp
Nothing should be changed for now. Because the DATA pin of the 433MHz RF Transmitter module is connected, stick to wiringPi’s GPIO 0. Press Ctrl + X to exit back to the terminal.
If codesend.cpp
was altered in any way, it needs to be compiled again with:
make codesend.cpp
Setting up the Arduino to receive RF signals
To set up an Arduino to receive RF signals, it needs to be connected to the 433MHz Receiver module. The Arduino also needs to be prepared and configured using the RC Switch library.
Connecting the Arduino to a 433MHz Receiver module
The 433MHz RF transmitter module is the rectangle (vs. square) module of the 433MHz RF Transmitter Receiver module pair.
As with the Transmitter module, the receiving range of the Receiving module can be extended by adding an antenna. The official 433 MHz Spiral Spring Helical Antennas can be used, or one can be made by coiling a 25 cm 1 mm solid wire and soldering it into the designated antennae hole.
The pins on the 433MHz RF Receiver module is once again clearly marked.
The receiver module has two DATA pins. As a rule, I like to always use the one closest to GND. Connect the breadboard wiring as follows:
- ATAD (DATA) to the D2 pin of the Arduino
- VCC (5V) to 5V pin of the Arduino
- GND to the GND pin of the Arduino
Preparing the Arduino to receive 433MHz RF signals
On the Arduino, the RC Switch library needs to be available in the Arduino IDE Libraries directory. To add this library to the Arduino IDE, select the latest version of the downloaded zip-file from Add .ZIP Library… from the Include Library option under the Sketch menu.
On a Linux system (including Raspbian) the following can be used to clone the latest version of the RC Switch library directly into the Arduino IDE libraries
folder:
sudo apt-get install git cd /home/pi/sketchbook/libraries git clone https://github.com/sui77/rc-switch.git
To prevent Java errors, rename the /rc-switch
directory to /rcswitch
:
mv /home/pi/sketchbook/libraries/rc-switch /home/pi/sketchbook/libraries/rcswitch
Connect the Arduino to the Arduino IDE and upload the following sketch to it:
/* RF_Sniffer Hacked from http://code.google.com/p/rc-switch/ by @justy to provide a handy RF code sniffer */ #include "RCSwitch.h" #include <stdlib.h> #include <stdio.h> RCSwitch mySwitch = RCSwitch(); void setup() { Serial.begin(9600); mySwitch.enableReceive(0); // Receiver on interrupt 0 > pin D2 } void loop() { if (mySwitch.available()) { int value = mySwitch.getReceivedValue(); if (value == 0) { Serial.print("Unknown encoding"); } else { Serial.print("Received "); Serial.print( mySwitch.getReceivedValue() ); Serial.print(" / "); Serial.print( mySwitch.getReceivedBitlength() ); Serial.print("bit "); Serial.print("Protocol: "); Serial.println( mySwitch.getReceivedProtocol() ); } mySwitch.resetAvailable(); } }
As is, this piece of coding makes the Arduino ‘sniff’ for RF code and if it receives it, it will be displayed on the serial monitor. The ‘bit length’ and ‘protocol’ variables will give more information about the code received. Don’t change this code too much until you are comfortable with Arduino code.
Other important things to notice here are the inclusion of the libraries and the setting up of the receiver pin D2.
Testing the communication
On the Raspberry Pi, go back to the /home/pi
directory:
cd /home/pi
A code send command can be issued from the terminal by using the absolute destination of codesend:
sudo ./433Utils/RPi_utils/codesend 1233434 sudo ./433Utils/RPi_utils/codesend 4321 sudo ./433Utils/RPi_utils/codesend 9876574
etc.
These three commands will send ‘1233434’, ‘4321’ and ‘9876674’ respectively. This should be visible on the Arduino Serial Monitor. If not, try to move the Receiver Arduino closer or further away while issuing the codesend
command.
Conclusion
The Raspberry Pi connecter to a Transmitted module can be used to transmit 433MHz RF signals to other devices. Devices such as remote controls, headphones, baby phones and other Raspberry Pis can receive these signals.