Twitter has taken the world by storm with its concept of tweets, ease of use and platform integration. Here we show how to post to Twitter using a Raspberry Pi.
Table of contents
Introduction to posting to Twitter from a Raspberry Pi
With more than 300 million users, Twitter is very popular and is available on almost all platforms.
The concept of a tweet is very similar to the older SMS (Short Message Service) but without some of its limitations. Hashtags and images can also be used. Tweets can be sent globally to multiple recipients at the same time and the costing structure is integrated into data usage.
Reasons for posting to Twitter from a Raspberry Pi range from a bit of fun to building a follower audience and sending information to an easily accessible platform.
- Get the Raspberry Pi 4B 4GB Starter Kit from Amazon.com
- Get the Raspberry Pi 4B 8GB Starter Kit from Amazon.com
Assumptions and requirements
For this post, a fully installed Raspberry Pi with Raspbian and a connection to the internet were used. Without a connected keyboard and screen, PuTTY and/or WinSCP can be used to do the testing and coding. On the social media side, access to a verified Twitter account with a working cell phone number is required.
Creating and configuring a Twitter App
To be able to post to Twitter from the Raspberry Pi, access to Twitter through their API will be required. For this, a Twitter App needs to be created.
To create a new Twitter App, open the Twitter Application Manager.
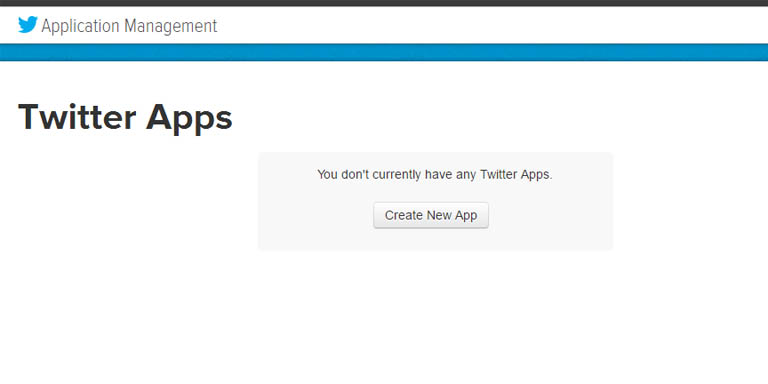
New Twitter Aps are created under Application Management.
After the app is created, set the access level to both read and write before generating an access token. The following information should now be available and noted down:
- Consumer Key (API Key)
- Consumer Secret (API Secret)
- Access Token
- Access Token Secret
Posting to Twitter using Python
To post to Twitter from a Raspberry Pi, additional Python libraries will be required. There are probably more, but this process was tested using Tweepy and Twython. Both these libraries are easy to install and fairly easy to use. Both Tweepy and Twython are installed using pip
.
The following terminal command can be used to install and update pip
:
sudo apt-get install python-pip sudo pip install --upgrade pip --index-url=https://pypi.python.org/simple/
Posting to Twitter using Tweepy
Tweepy is a very popular, easy-to-use, Python library for accessing the Twitter API. Although it is somewhat more complex to use than Twython (see below), it does have the advantage of being able to tweet images as well as text.
After installing pip, Tweepy is installed by using the following terminal command:
sudo pip install tweepy
To see if Tweepy has been installed, make sure it is on the list generated by:
pip list
There might be some difficulties installing Tweepy (and other pip
packages) starting from pip version 10. If this is the case, pip
needs to be downgraded again before trying to install Tweepy again. Pip can be downgraded using:
sudo -H pip install pip==8.1.1
Tweepy can be uninstalled using:
sudo pip uninstall tweepy
To use Tweepy, create a Python file in the desired directory:
nano tweepy-tweet.py
and add and adapt the following code to it before saving (this is the original script posted by Nikhil from NoDotCom.org):
#!/usr/bin/env python # -*- coding: utf-8 -*- import tweepy def get_api(cfg): auth = tweepy.OAuthHandler(cfg['consumer_key'], cfg['consumer_secret']) auth.set_access_token(cfg['access_token'], cfg['access_token_secret']) return tweepy.API(auth) def main(): # Fill in the values noted in step 2 here cfg = { "consumer_key" : "VALUE", "consumer_secret" : "VALUE", "access_token" : "VALUE", "access_token_secret" : "VALUE" } api = get_api(cfg) tweet = "Hello, world!" # tweet text (140 chars. max.) status = api.update_status(status=tweet) if __name__ == "__main__": main()
The values of VALUE and tweet should be replaced with your own (keep the quotation marks). The following terminal command can be used to run the script:
python tweepy-tweet.py
By modifying the code as follows, an image can also be tweeted:
api = get_api(cfg) tweet = "Hello, world!" # tweet text (140 chars. max.) image = "/home/pi/image.jpg" # image file destination #status = api.update_status(status=tweet) status = api.update_with_media(image, tweet)
Posting to Twitter using Twython
Twython is a pure Python wrapper for the Twitter API. It will work for Python 2.6+ and Python 3. Apart from tweeting, it can also do a few other things using the Twitter API.
After installing pip, Twython is installed by using the following terminal command:
sudo pip install twython
To use Twython, create a Python file in the desired directory:
nano twython-tweet.py
and add and adapt the following code to it before saving:
#!/usr/bin/env python # -*- coding: utf-8 -*- import sys from twython import Twython consumer_key = "VALUE" consumer_secret = "VALUE" access_token = "VALUE" access_token_secret = "VALUE" api = Twython(consumer_key,consumer_secret,access_token,access_token_secret) api.update_status(status=sys.argv[1])
The values of VALUE should be replaced with your own (keep the quotation marks). The following terminal command can be used to tweet:
python twython-tweet.py "Hello, world!"
This makes it easy to tweet directly from the terminal, a Bash script or another Python script.
Additional notes
Run Tweepy from a Bash script
Twython is straightforward to run from Bash, but Tweepy can be a bit more challenging. With the code below, the Bash script will forward the tweet and image directory to the modified Python script.
#!/bin/bash # Tweet (max. 140 characters) tweet="Hello, world!" # tweet text # Absolute directory and full file name of image full_image_path="/home/pi/image.jpg" python /home/pi/tweepy-tweet.py "$full_image_path;$tweet" # note that image and tweet is separated with a ";" for Python to work with
Include the following code at the top of the modified Python script to be able to handle the Bash strings:
import sys image_plus_tweet = ' '.join(sys.argv[1:]) # image directory and message (after ";") image = image_plus_tweet.split(";")[0] # strip everything after/including the ";". tweet = image_plus_tweet.split(';', 1)[-1] # strip everything before/including the ';'. if image == tweet: # meaning there is no image tweet = ""
Precautions
There are strict Twitter rules against what they consider to be spam. Using third-party applications and auto-posting might be flagged as spam. Twitter states the following:
“You are ultimately responsible for the actions taken with your account, or by applications associated with your account. Before authorizing a third-party application to access or use your account, make sure you’ve thoroughly investigated the application and understand what it will do.
If automated activity on your account violates the Twitter Rules or these Automation Rules, Twitter may take action on your account, including filtering your Tweets from search results or suspending your account.”
In order to prevent Twitter from flagging an account, make sure to use your Twitter account as a human too.
Conclusion
Twitter is a very popular social media platform and allows various forms of integration using its API. A Raspberry Pi can be used to post to Twitter using Twython and/or Tweepy.