The Raspberry Pi is a great stand-alone device that has the ability to use Bash scripts. Bash scripts allow multiple commands to be executed one by one. See how.
Table of contents
Introduction to Bash
Bash is a Unix shell and command language. It was written by Brian Fox as free software that has been used since 1989 as the default login shell for most Linux distributions. The Raspberry Pi OS (formerly known as Raspbian) is often used through the command line terminal.
Bash is used to execute commands. Bash scripts can also be created which allows a string of commands to be executed and/or basic forms of computer reasoning can be used.
Here we explain some very basic Bash and the basics of Bash script files, how to create and edit the files, set their privileges, where to store the files and how they are executed. Nano will be used as the text editor.
In the process, it will also show how to work with Linux directories, move, duplicate and remove files and how to create and remove directories. At the end of this post, a summary of the Bash commands used can be found.
- Get the Raspberry Pi 4B 4GB Starter Kit from Amazon.com
- Get the Raspberry Pi 4B 8GB Starter Kit from Amazon.com
Bash shell vs Bash scripts
Bash shell, or simply Bash, is a standard shell for Linux users. When using most Linux systems, including Raspbian, the operating system’s user commands can also be in the form of typed commands in the terminal. After each command is typed the Enter key is pressed for it to be executed. These commands and how they are interpreted by the system are called Bash. Commands include the system commands, execution of files (e.g. running programs, Python or Bash files or Bash scripts).
A Bash script consists of one or more Bash commands. Anything that can be executed in the command terminal can be placed in a Bash script. Each line of the Bash script represents an Enter key. Bash scripts are saved as .sh
files. Bash commands inside a script will be run sequentially.
Both Bash and Bash scripts can be scheduled to be executed by Cron.
To sudo or not to sudo
By default, Raspbian is configured to have one ‘superuser’. This is usually the user type that most Raspberry Pi users will be using (after logging in with pi
:raspberry
at the command prompt). By following the rest of this post it is assumed that the superuser is used.
Creating Bash scripts
Bash scripts are written as text files containing individual Bash commands. Each command is written as a separate line. Bash scripts are saved as .sh
files. Before creating the Bash script, make sure to be in the default working directory by using the following command:
cd /home/pi
Nano can be used to create files. By typing nano
and the name of the file to be created, Nano will either open the file if it already exists or create the a new file with that name if it does not:
nano helloworld.sh
On a fresh install, the helloworld.sh
file will most likely not exist and Nano will open a blank file.
To start editing the Bash script file, add the ‘shebang’ as the first line:
#!/bin/bash
The shebang is always added as the first line of a Bash script. The /bin/bash
part is the interpreter. This tells Raspbian that it is a Bash script and what shell to use — in this case Bash.
The hash sign (#
) at the beginning of the shebang tells Linux not to see it as code, but because it is the first line it will still attempt to identify the interpreter. A more traditional use for #
is as a commenting marker. In Linux, hashes are used to precede notes or to temporarily deactivate commands.
Now, add the following commenting marker to the second line of the script:
# This is a Bash script to say hello to the world.
To save the Bash script, press and hold Ctrl + X, and then press Y. For now, use the given name and directory for the file to be saved in (i.e. press Enter).
The directory structure
In order to keep things organised, files on an operating system are stored in directories. The principle of a directory is similar to a folder in other operating systems. On the Raspberry Pi running Raspbian, the default user directory is /home
followed by the username, in this case as /pi
. Each time the system boots, Raspbian will start in this (/home/pi
) directory. When the user is inside /home/pi
directory it will not show on the terminal.
When a command is given, Raspbian will assume to use the directory the user is currently in. At any time, the user can use the pwd
terminal command to see where the command will be triggered from.
After the Bash script has been saved, the user will be returned to the terminal. By default, Nano will prompt to save files in the current working directory. In this case, it was /home/pi
.
To list all the files in this directory, the dir
terminal command can be used. This will show the newly created helloworld.sh
.
Creating new directories
New directories can be created to organise groups of files neatly. For Bash script files, any directory within /home/pi
can be used, but many Linux users like to use a /bin
directory.
To create a new directory the mkdir
terminal command, followed by the directory name, is used. Use the following terminal command to create a /bin
directory inside the /home/pi
directory:
mkdir /home/pi/bin
By using the dir
command again from the /home/pi
directory, the /bin
directory, together with helloworld.sh
file, will be listed.
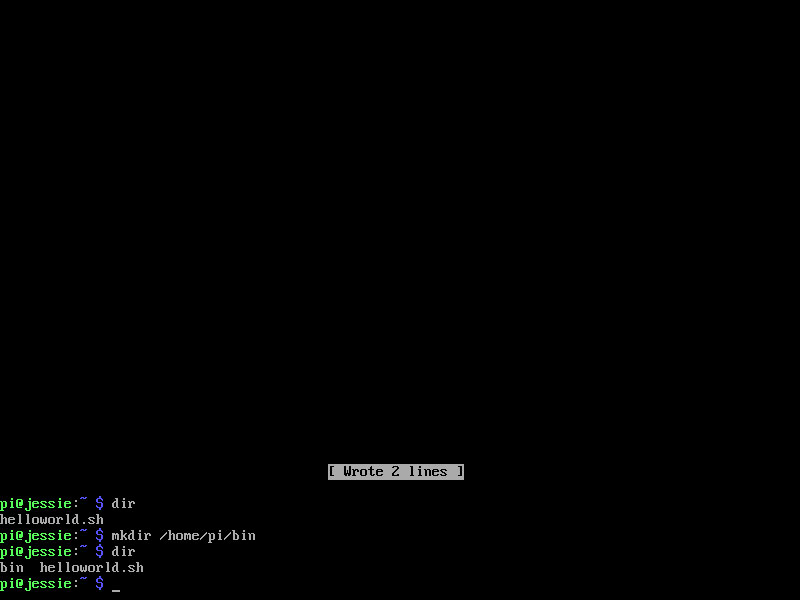
To see the listed contents of a directory, use the ‘dir’ terminal command. In this case, directories will also be listed.
Moving to a directory
To move to a directory (i.e. change the active directory), the cd
command, followed by name of the directory is used. To move to the newly created /bin
directory, the cd
command is used as follows:
cd /home/pi/bin
By using the cd
command on its own (i.e. without the name of a directory), the user will be taken back to the default user directory (in this case, /home/pi
).
The absolute directory structure
To prevent confusion, rather get into the habit of using the full, or absolute directory structure. Directory-related commands (e.g. dir
, mkdir
, cd
, mv
, cp
& rm
) can also be used with the relative directory structure.
If for example the mkdir
command was used with /bin
instead of bin
(note the forward slash), Raspbian would have attempted to create a /bin
directory in the root of the operating system and not /home/pi/bin
. By using the absolute directory structure the need to keep track of the current user directory also becomes less important.
Moving or copying files between directories
Files can be moved or copied from one directory to another by using the mv
or cp
commands. The mv
command will copy the file to a target directory and will automatically delete it from the source directory — similar to cut and paste. The cp
command will copy the file to a target directory without deleting the if from the source directory.
Both these commands are followed by the file to be moved or copied (including the target directory) and the destination directory:
mv /targetdirectory/file.sh /destinationdirectory
or
cp /targetdirectory/file.sh /destinationdirectory
To move helloworld.sh
to the newly created bin
directory, the command is used as follows:
mv /home/pi/helloworld.sh /home/pi/bin
To see if the file has been moved, use the following two dir
commands:
dir /home/pi dir /home/pi/bin
Editing files
Now that the Bash script has been moved to the /home/pi/bin
directory, we can continue to update the Bash file. To open an existing Bash script file, the nano
terminal command can be used again:
nano /home/pi/bin/helloworld.sh
Fo illustration purposes the echo
command will be used, but any Bash command can be used. In Bash, the echo
command is used to print desired text on the screen. Add the following line to the Bash script:
echo "Hello, world..."
Here, the echo
command will display the text between opening and closing quotation marks on the screen — Hello, world… The quotation marks are to identify the beginning and the end of the text to be displayed and will not be ‘echoed’.
Press and hold Ctrl + X, and then press Y to save the file again.
Running Bash scripts
Now that the Bash script contains the updated commands, it is ready to be executed. Bash script files can either be executed from the terminal or from Cron using the bash command. To run the script, use the bash
terminal command as follows:
bash /home/pi/bin/helloworld.sh
Removing/deleting a file or directory
When files or directories need to be removed, the rm
and rmdir
commands are used. The rm
command is used to delete a file and the rmdir
command is used to delete a directory. Similarly to all the other directory-related commands mentioned earlier, these commands are trailed by either the file name or the directory to be deleted.
To delete helloworld.sh
the rm
command can be used as follows:
rm /home/pi/bin/helloworld.sh
The rmdir
command is slightly different from the other directory-related commands in that only empty directories can be deleted and that the user cannot be inside the directory while attempting to delete it.
The cd
command can be used to move to a different directory before it is deleted. To delete the /home/pi/bin
directory the /rmdir
command can be used as follows:
cd /home/pi rmdir /home/pi/bin
Raspbian command line summary
Create and/or Edit a file:
nano /home/pi/bin/helloworld.sh
Create a directory:
mkdir /home/pi/bin
Move a file:
mv /home/pi/helloworld.sh /home/pi/bin
Duplicate a file (copy/paste):
cp /home/pi/helloworld.sh /home/pi/bin
Remove a file:
rm /home/pi/helloworld.sh
Remove a directory (needs to be empty first):
rmdir /home/pi/bin
Execute a Bash script:
bash /home/pi/bin/helloworld.sh
Conclusion
Bash is used to execute commands and Bash scripts allow a string of commands to be executed. Here we showed how to create and edit Bash script files, set their privileges, where to store the files and how they are executed using Nano. The process also showed how to work with Linux directories, move, duplicate and remove files and how to create and remove directories.