A handy feature of WordPress is its shortcode functionality. This post will go through some basic principles of creating and using shortcodes in WordPress.
Table of contents
Introduction to using shortcodes in WordPress
WordPress plugin and theme developers often use shortcodes to incorporate functionality for their users.
Shortcodes come in many shapes and sizes, but ultimately they are like shortcuts to display larger pieces of code. After a WordPress shortcode is created, it can be used in the Post/Page Editor and/or in WordPress theme template files.
In its simplest form, a WordPress shortcode is a piece of WordPress code that represents another piece of code. It is easily identified by being encapsulated in square brackets (e.g. [shortcode_name]
).
Although shortcodes are meant to be used in the WordPress Post/Page editor, they can also be used in theme template files. Their content is shown on the front end.
The code that is represented by the shortcode can be in the form of HTML, CSS, JavaScript and/or PHP.
WordPress itself has a couple of handy, built-in shortcodes, but additional shortcodes can also be created using plugins and/or theme functions.
- Get the LG 22MK430H-B 21.5″ Full HD Monitor from Amazon.com
- Get the Corsair K95 RGB Platinum XT Mechanical Gaming Keyboard from Amazon.com
- Get the ROCCAT Burst Pro PC Gaming Mouse from Amazon.com
Why use shortcodes in WordPress
WordPress shortcodes have many uses. Apart from shortening pieces of code, shortcodes are also often used to ‘recycle’ code, add WordPress functionality to where it is used, to centralise code and to keep complex code away from inexperienced users.
Pieces of code (e.g. HTML code showing a message) that will be used on various Posts and/or Pages can be converted into a shortcode. This allows the code to be reused without having to retype it over and over again. If the piece of code (e.g. the message) needs to be changed at some point, it only needs to be done in one place.
Complex, large and/or functional pieces of code can be written with more complex PHP or JavaScript programming languages. These pieces of code can be converted into a shortcode, bringing it, together with its functionality to, for example, the Post Editor.
The shortcode will also shorten the code significantly, preventing unnecessary clutter in the Post Editor.
Creating custom WordPress shortcodes
Custom WordPress shortcodes can be created using the Shorcoder plugin and/or by using theme functions.
Sample code
As an example, let’s say we want to add and reuse the following ‘Follow me on social media!’ message:
The HTML and CSS code for this message looks as follows (inline CSS was used to make things less complicated):
<div style="text-align: center; background: red; color: white; margin: 20px; padding: 10px;">Follow me on social media!</div>
Creating custom shortcodes using the Shortcoder plugin
The easiest way to create custom shortcodes with WordPress is by using a plugin. There might be a couple of plugins available, but a popular free choice is the Shortcoder plugin created by Aakash Chakravarthy.
The Shortcoder plugin is a back end plugin that is used to create, manage and update shortcodes without being code-savvy. At the time of writing Shortcoder was at version 5.3, had more than 60 000 active installs and an average rating of 5 out of 5 stars.
After installing and activating the Shortcoder plugin from the WordPress Plugins section, its settings will be available under the Shortcoder menu.
Using the sample code described earlier, Create a new shortcode, name it, and add (or paste) the code to the Shortcode content section.
Code can either be added using the Visual editor and/or the Text editor feature. The shortcode is saved by clicking the Create shortcode button at the bottom.
Multiple shortcodes can be created. Shortcoder will make sure that there are no duplicate shortcode names.
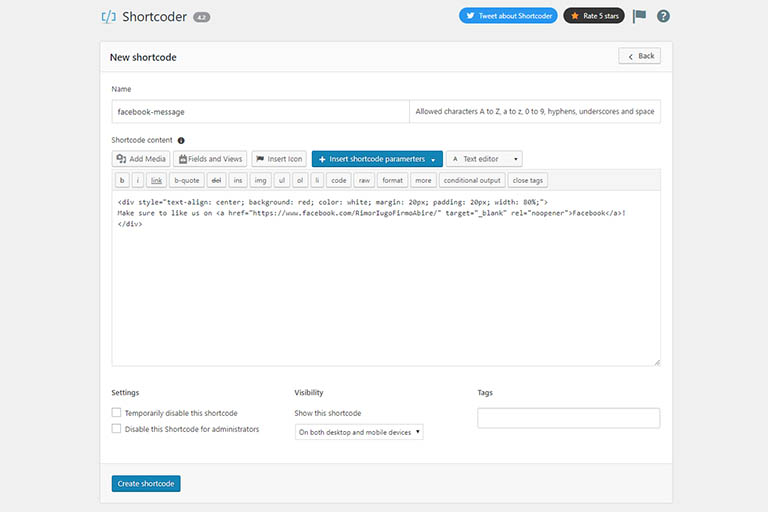
An example of using the Shortcoder plugin to create a WordPress shortcode. The sample code shown earlier was added to the Shortcoder Visual Editor. Note that the editor was set to the Text editor.
By using, for example, ‘my_social_media_message’ as the name of the shortcode, Shortcoder will create a shortcode named .
Creating custom shortcodes using theme functions
For those that don’t like plugins, like to have more control and/or is a bit more code-savvy, shortcodes can also be created manually by using PHP and theme functions.
To create a shortcode using functions, the shortcode callback (or output) function together with the WordPress add_shortcode()
function is added to the main plugin file or the (child) theme’s functions.php
file.
To create a callback function to output the example code/message described earlier, the following code needs to be added before the closing ?>
tag of the functions.php
file.
The final PHP code will look as follows:
add_action( 'init', 'bts_register_shortcodes' ); function bts_register_shortcodes() { // Registering shortcodes using the add_shortcode() function. // [my_social_media_message] add_shortcode( 'my_social_media_message', 'bts_social_media_message' ); } /** * Callback function that returns HTML code as a string */ function bts_social_media_message() { return '<div style="text-align: center; background: red; color: white; margin: 20px; padding: 10px;">Follow me on social media!</div>'; }
To break things down, the first step is to create a callback function to output the example code/message described earlier. The content of this function is what is displayed when the shortcode is called:
function bts_social_media_message() { return '<div style="text-align: center; background: red; color: white; margin: 20px; padding: 10px;">Follow me on social media!</div>'; }
where bts_social_media_message
is the name of the callback function. Note that this function needs to return
the code (i.e. not echo
it). In this case, the code is encapsulated in single quotation marks to indicate that it is a string.
In order to prevent clashes and errors, the callback function name needs to be unique. It is good practice to add a prefix to the function name. In this case, the prefix bts_
was used.
Secondly, to be able to use the output of bts_social_media_message()
as a shortcode, it needs to be registered with WordPress. This is done with the WordPress add_shortcode()
function.
According to the WordPress codex, the WordPress add_shortcode()
function takes two arguments/parameters:
- The shortcode tag that is to be used as the identifying name of the shortcode (in this case,
'my_social_media_shorcode'
) - The name of the function handling the output of the shortcode (i.e. the callback function — in this case,
'bts_social_media_message'
)
As with the callback function, the shortcode tag also needs to be unique. This is so they don’t interfere with other shortcodes included by WordPress core, themes or plugins.
If there is another shortcode output function with the same function name WordPress will throw a critical error and if there is another shortcode with the same tag, the latest one will be used.
To continue with the example, this is what the add_shortcode()
function should look like:
add_shortcode( 'my_social_media_message', 'bts_social_media_message' );
This tells WordPress that if the shortcode [my_social_media_message]
is called, the contents of the bts_social_media_message()
function is to be returned.
It is good practice to call the add_shortcode()
function before the callback function. It is also good practice to describe the callback function for future use:
// Registering shortcodes using the add_shortcode() function. add_shortcode( 'my_social_media_message', 'bts_social_media_message' ); /** * Callback function that returns HTML code as a string */ function bts_social_media_message() { return '<div style="text-align: center; background: red; color: white; margin-bottom: 20px;">Follow me on social media!</div>'; }
Thirdly, multiple shortcode functions can be created this way, but when there are more than one, all the shortcodes can be registered better using one shortcode callback function:
function bts_register_shortcodes() { // Registering shortcodes using the add_shortcode() function. add_shortcode( 'my_social_media_message', 'bts_social_media_message' ); add_shortcode( 'my_shortcode_2', 'bts_my_shortcode_2' ); add_shortcode( 'my_shortcode_3', 'bts_my_shortcode_3' ); // etc. }
where bts_register_shortcodes
is the name of the shortcode callback function. Because this is a custom function, the name also needs to be unique, so the prefix bts_
was used.
Lastly, in order to give WordPress enough time to run other required code, it is recommended to register shortcodes at the time WordPress is initiated or after the theme has been set up. This is done using the WordPress init()
or the WordPress after_setup_theme()
action hooks.
The after_setup_theme()
function runs before the init()
function and is generally used to initialize theme settings/options before a user is authenticated.
The init()
function runs after a user is authenticated, but before any headers are sent. According to the WordPress code reference, “most of WP is loaded at this stage, and the user is authenticated.”
To use the init()
function action hook, the following code is added before the register_shortcodes()
function:
add_action( 'init', 'bts_register_shortcodes');
To use the register_shortcodes()
function instead, simply replace 'init'
with 'after_setup_theme'
.
The final shortcode PHP code will look as follows:
add_action( 'init', 'bts_register_shortcodes'); function bts_register_shortcodes() { // Registering shortcodes using the add_shortcode() function. add_shortcode( 'my_social_media_message', 'bts_social_media_message' ); } /** * Callback function that returns HTML code as a string */ function bts_social_media_message() { return '<div style="text-align: center; background: red; color: white; margin: 20px; padding: 10px;">Follow me on social media!</div>'; }
Inserting shortcodes into WordPress
After a shortcode has been created, it can either be used in the WordPress Post/Page Editor or inside theme template files.
Inserting shortcodes with the WordPress Editor
The most common (and easiest) way to add a shortcode to WordPress is by adding the shortcode into the WordPress Post/Page Editor.
If the Shortcoder plugin is installed, created shortcodes can either be copied and pasted or inserted into content areas using the Shortcoder button at the top of the WordPress Post Editor.
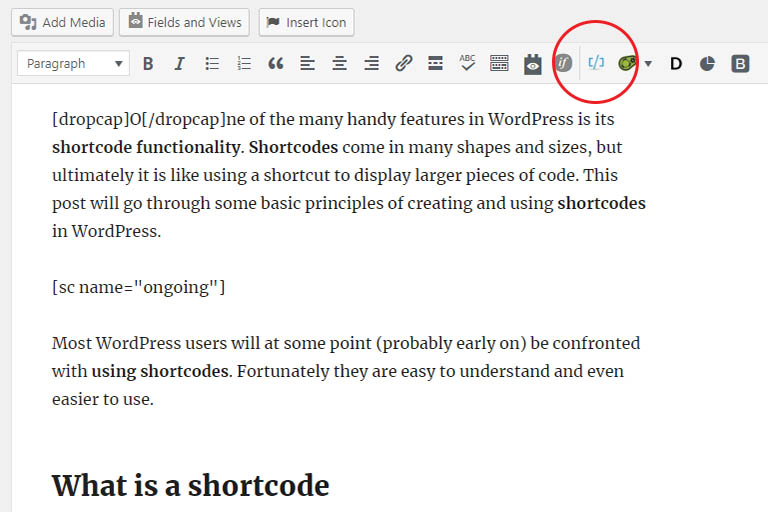
After the Shortcoder plugin has been installed, a Shortcoder button will be visible on the top of the WordPress Post/Page Editor. In this case, a shortcode, named “ongoing” was added to the post.
When functions were used to create a shortcode, the shortcode tag name (e.g. 'my_social_media_message'
) is used as the name of the shortcode. The shortcode itself will be the name of the tag encapsulated in square brackets (e.g. [my_social_media_message]
).
Note that the content of the shortcode will not show in the Editor. It will only be visible after the post/page was saved, refreshed and previewed.
Inserting shortcodes into WordPress theme template files
For areas of a website which is not accessible through a text editor, e.g. the website footer area, shortcodes can be added using PHP. The following code will add the earlier example:
echo do_shortcode('[my_social_media_message]');
or
echo do_shortcode('');
Conclusion
A shortcode acts like a little envelope for more complex code, allows the addition of code to the WordPress Editor, or can be used for repeating code.
Shortcodes in WordPress can either be created using the Shortcoder plugin and/or by adding shortcode functions to theme or plugin files.