Pages are one of WordPress' main post types but don't have a customisable excerpt field. Here we show how to enable excerpts for Pages in WordPress.
Table of contents
Introduction to WordPress excerpts
WordPress uses excerpts as a short introduction for Posts (the Post Post type). Excerpts are commonly used on front, archive and search pages to interest readers without needing to display the full content of the post and to make them want to read the post further. They can also be used inside a post to highlight the key point or introduce the content.
Pages (the Page Post type) do not have excerpts enabled by default. WordPress theme developers might want to add excerpts to the Page Post type for the following reasons:
- To be displayed as a loop on the front, archive and search pages
- To be able to use/manipulate its content in the page.php template file
In the case where Pages are added to loop functions and it does not contain a manual excerpt, the first (usually 55) words of the content will be used by WordPress to generate its own excerpt. By adding text to the excerpt meta box, WordPress will use that one instead.
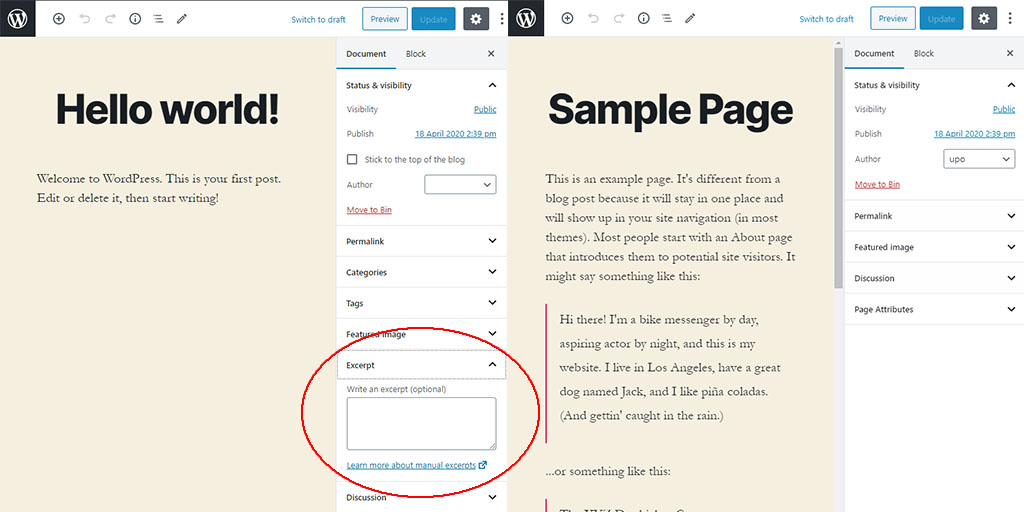
Side-by-side screens of the default Post (left) and default Page Add new/Edit sections. Note that the Post Edit screen has an excerpt meta box (red circle) and the Page Edit Screen does not.
- Get the Corsair K95 RGB Platinum XT Mechanical Gaming Keyboard from Amazon.com
- Get the ROCCAT Burst Pro PC Gaming Mouse from Amazon.com
- Get the LG 22MK430H-B 21.5″ Full HD Monitor from Amazon.com
Enabling custom excerpts for WordPress Pages
To enable custom excerpts for Pages, post type support for page excerpts needs to be added. This, and the other code snippets mentioned later, can either be done by updating the functions.php
file of the (child) theme or a custom site-specific plugin.
Post type support can be added using WordPress’ add_post_type_support()
function.
Using the WordPress add_post_type_support() function
WordPress has a function called add_post_type_support()
. According to the WordPress code reference, the aim of the add_post_type_support()
function is to register certain features for a certain post type.
It goes further by saying that all core WordPress features are directly associated with a functional area of the edit screen, such as the editor or a meta box. Apart from the ‘excerpt’, these features also include ‘title’, ‘editor’, ‘comments’, ‘revisions’, ‘trackbacks’, ‘author’, ‘page-attributes’, ‘thumbnail’, ‘custom-fields’ and ‘post-formats’. The default post types in WordPress are ‘post’, ‘page’, ‘attachment’, ‘revision’ and ‘nav_memu_item’.
In its simplest form, the add_post_type_support()
function will need two arguments/parameters, the post type and the feature that needs to be added. To activate custom excerpts for Pages, the post type is page
and the feature is excerpt
:
// Adding the excerpt feature or the Post post type add_post_type_support( 'page', 'excerpt' );
Once the theme or plugin code is updated and saved, new and existing Pages will show an excerpt meta box.
Using WordPress Page excerpts
Adding custom excerpts to Pages will often be enough for WordPress to start using them automatically in the default areas of the rest of the site. For WordPress websites that make frequent use of Pages, developers might also want to add Page excerpts to additional areas.
After excerpts for Pages have been activated, Page excerpts can be called in exactly the same way as Post excerpts, namely using WordPress PHP functions or using shortcodes.
Calling Post and Page excerpts using WordPress PHP functions
Posts and Page excerpts can be called by one of two WordPress PHP functions:
the_excerpt()
get_the_excerpt()
By looking at the WordPress code reference, the major difference between these two functions is that get_the_excerpt()
collects the raw excerpt from the WordPress database and the_excerpt()
gives a filtered version of get_the_excerpt()
— most noticeably adding paragraph <p></p>
tags to the excerpt.
The the_excerpt()
function also echoes the excerpt whereas get_the_excerpt()
returns it.
The default WordPress loop for index.php
, archive.php
and search.php
pages will display excerpts in a way similar to this:
if ( have_posts() ) { while ( have_posts() ) { the_excerpt(); // rest of page/post content here } // end while } // end if
This will display the excerpt encapsulated in paragraph <p></p>
tags.
To have a little more control over the excerpt, e.g. manipulate its content, use different tags or add classes and/or ids, the get_the_excerpt()
function can be used:
if ( have_posts() ) { while ( have_posts() ) { $excerpt = get_the_excerpt(); // do something with $excerpt echo '<p class="excerpt-class">' . $excerpt . '</p>'; // rest of post content here } // end while } // end if
Autogenerated excerpts in WordPress
If the content in the excerpt meta box is left empty, both the_excerpt()
and get_the_excerpt()
functions will generate their own excerpt based on the content of the post or page. This autogenerated excerpt will, by default, have 55 words, and ellipses, in the form of […]
, will be added at the end. This is also referred to as the “more” section of the excerpt.
According to WordPress, the function responsible for autogenerating post and page excerpts is wp_trim_excerpt()
.This behaviour of this function can be altered by preventing WordPress from displaying an empty excerpt, changing the excerpt length, and/or changing the “more” section of the excerpt.
Prevent WordPress from displaying an empty excerpt
To prevent WordPress from generating its own excerpt, the has_excerpt()
function can be used. This function will return a true or false value depending on whether the Page (or Post) has an excerpt or not:
if ( ! has_excerpt() ) { echo ''; } else { the_excerpt(); }
or
if ( ! has_excerpt() ) { echo ''; } else { echo get_the_excerpt(); }
Changing the excerpt length in WordPress
By default, the maximum excerpt length in WordPress is 55 words. This can be changed to any number of words by using the excerpt_length
filter:
// Return an updated excerpt length function bts_custom_excerpt_length( $length ) { return 20; } add_filter( 'excerpt_length', 'bts_custom_excerpt_length', 999 );
According to the WordPress developer resources, the priority of the excerpt_length
filter needs to be set to something very late, e.g. 999, otherwise, the default WordPress filter on this function will run last and override what is set here.
In this case, the bts_custom_excerpt_length()
function will return 20 — meaning that the maximum length of the excerpt will now be 20 words.
Changing the “more” section of the excerpt
The “more” section of an auto-generated excerpt in WordPress is created by using the excerpt_more
filter. According to the WordPress developer resources, it is applied after the wp_trim_excerpt()
function and returns […]
.
Practically, a function that generates the “more” section hooked into the excerpt_more
filter will look something like this:
// Returns the default WordPress "more" section function bts_excerpt_more( $more ) { return '[…]'; } add_filter( 'excerpt_more', 'bts_excerpt_more');
In this case, a function named bts_excerpt_more()
was used. Its return value can be changed to whatever the “more” section needs to be.
Removing the “more” section of the excerpt
To remove the “more” section of the excerpt completely, the excerpt_more
filter needs to be supplied with an empty return string. This can either be done by changing the return value of the filtered function, or by filtering WordPress’ own empty function:
// Returns an empty "more" section function bts_excerpt_more( $more ) { return ''; } add_filter( 'excerpt_more', 'bts_excerpt_more');
or, simply
add_filter( 'excerpt_more', '__return_empty_string' );
In this case, the built-in WordPress function __return_empty_string()
was supplied to the excerpt_more
filter to return an empty string.
Adding a link to the “more” section of the excerpt
The “more” section of the excerpt can also be used to supply a link to the Post or Page:
// Returns a link to the Post/Page function bts_excerpt_more( $more ) { global $post; return '... <a class="read-more-class" href="'. get_permalink($post->ID) . '">' . 'Read more' . '</a>'; } add_filter( 'excerpt_more', 'bts_excerpt_more');
Here, the link to the post is generated using the get_permalink()
function — which needs the Post or Page ID. The post/page ID is obtained using $post->ID
.
The same reasoning can be applied to add links by using the get_the_excerp()
function code seen earlier.
Creating loop code to only display Pages
$args = array( 'post_type' => array( 'page' ), 'posts_per_page' => 10, ); // The Query $the_query = new WP_Query( $args ); // The Loop if ( $the_query->have_posts() ) { while ( $the_query->have_posts() ) { $the_query->the_post(); echo '<h3p>'. get_the_title() . '</h3p>'; the_excerpt(); } /* Restore original Post Data */ wp_reset_postdata(); } else { // no posts found }
The 'posts_per_page'
argument will set the number of pages. In the code above it was set to 10.
Conclusion
There might be many reasons why WordPress theme developers want to add custom excerpts to Pages. After custom excerpts for Pages have been activated, Post or Page excerpts can be shown using WordPress PHP functions or shortcodes. Post and Page excerpts can also be manipulated in various ways.